Wallet¶
Class Name | Wallet |
---|---|
Extends | Logger |
Source | wallet.ts |
Examples | wallet.spec.ts |
The Wallet
module is a wrapper for the MultiSigWallet and allows to create wallets, manage owners and execte transactions with it.
One of the common use cases is setting confirmation count to 1 (which is the default value). This basically makes the wallets a multiowned account, where all parties are able to perform transactions on their own.
The Wallet
module is bound to a wallet contract instance. This instace can be loaded, which requires an existing MultiSigWallet contract and a user, that is able to work with it:
wallet.load('0x0123456789012345678901234567890123456789');
If no wallet exists, a new wallet can be created with:
// account id, that creates the wallet
const accountId = '0x0000000000000000000000000000000000000001';
// account, that will be able to manage the new wallet
const manager = '0x0000000000000000000000000000000000000001';
// wallet owners
const owners = [
'0x0000000000000000000000000000000000000001',
'0x0000000000000000000000000000000000000002',
];
await wallet.create(accountId, manager, owners);
The last example creates a wallet
- with the account
0x0000000000000000000000000000000000000001
(used asaccountId
) - that can be managed (adding/removing owners) by
0x0000000000000000000000000000000000000001
(used asmanager
) - that allows the accounts
0x0000000000000000000000000000000000000001
and0x0000000000000000000000000000000000000002
to perform transactions (used asowners
)
Creating wallets via the Wallet
module loads them to the module as well, so there is no need to call the load
function after that.
So now we have a valid and working wallet loaded to our module and start performing calls. Let’s say, we have an instance of an owned contract and want to transfer its ownership, we can do:
const accountId = '0x0000000000000000000000000000000000000001';
const newOwner = '0x0000000000000000000000000000000000000002';
await wallet.submitTransaction(ownedContract, 'transferOwnership', { from: accountId, }, newOwner);
Looks pretty simple, but comes with a few things to consider:
accountId
is the identity or account, that performs the transaction from the “outside perspective” (if you look into a chain explorer like the evan.network Test-Explorer you’ll see, that identity or accountaccountId
is actually performing the transaction and not the wallet contract)accountId
pays the gas cost for the transaction (obviously, when considering the last point)- from the perspective of the target contract
ownedContract
, the wallet contract (either loaded or created beforehand) is performing the transaction - taken our example transaction
transferOwnership
, the wallet contract has to be the current owner and not the identity or accountaccountId
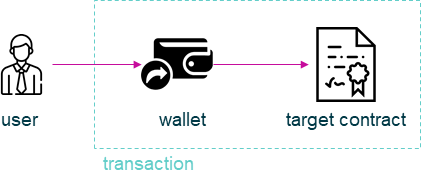
transaction flow in wallet based transactions
An implementation of an Executor
module, that uses a wallet for contract execution, has been created as ExecutorWallet
. Because the profiles are (externally owned) account based and many modules rely on profiles for data encryption and decryption, the ExecutorWallet
module should be used with care, when dealing with operations, that require en- or decryption.
constructor¶
new Wallet(options);
Creates a new Wallet instance.
Parameters¶
options
-WalletOptions
: options for Wallet constructor.contractLoader
-ContractLoader
:ContractLoader
instancedescription
-Description
:Description
instanceeventHub
-EventHub
:EventHub
instanceexecutor
-Executor
:Executor
instancenameResolver
-NameResolver
:NameResolver
instance
Returns¶
Wallet
instance
Example¶
const wallet = new Wallet({
contractLoader,
description,
eventHub,
executor,
nameResolver,
});
= Contract Management =¶
create¶
wallet.create(accountId, manager, owners);
Create a new wallet contract and uses it as its wallet contract.
Parameters¶
accountId
-string
: identity or account, that creates the walletmanager
-string
: identity or account, that will be able to manage the new walletowners
-string[]
: wallet ownersconfirmations
-number
(optional): number of confirmations required to complete a transaction, defaults to1
Returns¶
Promise
returns void
: resolved when done
load¶
wallet.load(contractId[, walletType]);
Load wallet contract from address and uses it as its wallet contract.
Parameters¶
contractid
-string
: a wallet contract addresswalletType
-string
(optional): wallet contract type, defaults toMultiSigWallet
Returns¶
Promise
returns void
: resolved when done
Example¶
wallet.load('0x0123456789012345678901234567890123456789');
= Transactions =¶
submitTransaction¶
wallet.submitTransaction(target, functionName, inputOptions[, ...functionArguments]);
Submit a transaction to a wallet, as required is fixed to 1, this will immediately execute the transaction.
Parameters¶
target
-any
: contract of the submitted transactionfunctionName
-string
: name of the contract function to callinputOptions
-any
: currently supported: from, gas, value, event, getEventResult, eventTimeout, estimate, forcefunctionArguments
-any[]
: optional arguments to pass to contract transaction
Returns¶
Promise
returns any
: status information about transaction
Example¶
await wallet.submitTransaction(testContract, 'transferOwnership', { from: accounts[0], }, accounts[1]);
= Account Management =¶
addOwner¶
wallet.addOwner(accountId, toAdd);
Function description
Parameters¶
accountId
-string
: identity or account with management permissions on wallettoAdd
-string
: identity or account to add as an owner
Returns¶
Promise
returns void
: resolved when done