ExecutorWallet¶
Class Name | ExecutorWallet |
---|---|
Extends | Executor |
Source | executor-wallet.ts |
Examples | executor-wallet.spec.ts |
The ExecutorWallet
module is designed to cover basically the same tasks as the Executor
module. While the last one performs the transactions directly with an identity or account, that is given as inputOptions, the ExecutorWallet
module wraps those transactions by submitting them to a configured wallet contract.
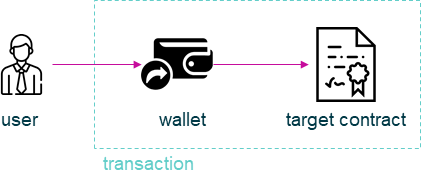
transaction flow in wallet based transactions
The wallet is a smart contract and the identity or account, that performs the transactions against the target contracts on the blockchain. Transactions are wrapped by using the Wallet
module, see details on how to transactions are performed internally at the documentation page of the Wallet
module.
Because transactions are performed via the Wallet
module, they have the same limitations in regards to en- and decryption as described in the introduction section of the Wallet
.
constructor¶
new ExecutorWallet(options);
Creates a new ExecutorWallet
instance.
The ExecutorWallet allows to pass the defaultOptions
property to its constructor. This property contains options for transactions and calls, that will be used if no other properties are provided in calls/transactions. Explicitly passed options always overwrite default options.
Parameters¶
options
-ExecutorWalletOptions
: options for ServiceContract constructor.accountId
-string
: account, that is used for making transactions against wallet contractconfig
-any
: configuration object for the executor instanceeventHub
-EventHub
:EventHub
instancesigner
-SignerInterface
:SignerInterface
instancewallet
-Wallet
:Wallet
instance with a loaded wallet contractweb3
-Web3
:Web3
instancedefaultOptions
-any
(optional): default options for web3 transactions/callslog
-Function
(optional): function to use for logging:(message, level) => {...}
logLevel
-LogLevel
(optional): messages with this level will be logged withlog
logLog
-LogLogInterface
(optional): container for collecting log messageslogLogLevel
-LogLevel
(optional): messages with this level will be pushed tologLog
Returns¶
ExecutorWallet
instance
Example¶
const executor = new ExecutorWallet({
accountId,
config,
eventHub,
signer,
wallet,
web3
});
init¶
executor.init(name);
initialize executor
Parameters¶
options
-any
: object with the property “eventHub” (of the type EventHub)eventHub
-EventHub
: The initialized EventHub Module.
Returns¶
void
.
executeContractCall¶
executor.executeContractCall(contract, functionName, ...args);
run the given call from contract
note, that if a from is used, this from is replaced with the wallets address
Parameters¶
contract
-any
: the target contractfunctionName
-string
: name of the contract function to call...args
-any[]
: optional array of arguments for contract call. if last arguments is {Object}, it is used as the options parameter
Returns¶
Promise
resolves to any
: contract calls result.
Example¶
const greetingMessage = await runtime.executor.executeContractCall(
contract, // web3.js contract instance
'greet' // function name
);
executeContractTransaction¶
executor.executeContractTransaction(contract, functionName, inputOptions, ...functionArguments);
execute a transaction against the blockchain, handle gas exceeded and return values from contract function,
note, that a from passed to this function will be replaced with the wallets address and that transactions, that transfer EVEs to a target identity or account, will be rejected
Parameters¶
contract
-any
: contract instancefunctionName
-string
: name of the contract function to callinputOptions
-any
: options objectfrom
-string
(optional): The address the call “transaction” should be made from.gas
-number
(optional): The amount of gas provided with the transaction.event
-string
(optional): The event to wait for a result of the transaction,getEventResult
-function
(optional): callback function which will be called when the event is triggered.eventTimeout
-number
(optional): timeout (in ms) to wait for a event result before the transaction is marked as errorestimate
-boolean
(optional): Should the amount of gas be estimated for the transaction (overwritesgas
parameter)force
-string
(optional): Forces the transaction to be executed. Ignores estimation errorsautoGas
-number
(optional): enables autoGas 1.1 ==> adds 10% to estimated gas costs. value capped to current block.
...functionArguments
-any[]
: optional arguments to pass to contract transaction
Returns¶
Promise
resolves to: no result
(if no event to watch was given), the event
(if event but no getEventResult was given), the
value returned by getEventResult(eventObject).
Because an estimation is performed, even if a fixed gas cost has been set, failing transactions are rejected before being executed. This protects users from executing transactions, that consume all provided gas and fail, which is usually not intended, especially if a large amount of gas has been provided. To prevent this behavior for any reason, add a force: true
to the options, though it is not advised to do so.
To allow to retrieve the result of a transaction, events can be used to receive values from a transaction. If an event is provided, the transaction will only be fulfilled, if the event is triggered. To use this option, the executor needs to have the eventHub
property has to be set. Transactions, that contain event related options and are passed to an executor without an eventHub
will be rejected immediately.
Example¶
const accountId = '0x...';
const greetingMessage = await runtime.executor.executeContractTransaction(
contract, // web3.js contract instance
'setData', // function name
{ from: accountId, }, // perform transaction with this account
123, // arguments after the options are passed to the contract
);
Provided gas is estimated automatically with a fault tolerance of 10% and then used as gas limit in the transaction. For a different behavior, set autoGas in the transaction options:
const greetingMessage = await runtime.executor.executeContractTransaction(
contract, // web3.js contract instance
'setData', // function name
{ from: accountId, autoGas: 1.05, }, // 5% fault tolerance
123, // arguments after the options are passed to the contract
);
or set a fixed gas limit:
const greetingMessage = await runtime.executor.executeContractTransaction(
contract, // web3.js contract instance
'setData', // function name
{ from: accountId, gas: 100000, }, // fixed gas limit
123, // arguments after the options are passed to the contract
);
Using events for getting return values:
const contractId = await runtime.executor.executeContractTransaction(
factory,
'createContract', {
from: accountId,
autoGas: 1.1,
event: { target: 'FactoryInterface', eventName: 'ContractCreated', },
getEventResult: (event, args) => args.newAddress,
},
);
executeSend¶
executor.executeSend(options);
sending funds is not supported by the walled based executor, use a regular Executor for such tasks
createContract¶
executor.createContract(contractName, functionArguments, options);
creating contracts directly is not supported by the walled based executor, use a regular Executor for such tasks