Profile¶
Class Name | Profile |
---|---|
Extends | Logger |
Source | profile.ts |
Examples | profile.spec.ts |
A users profile is its personal storage for - contacts - encryption keys exchanged with contacts - an own public key for exchanging keys with new contacts - bookmarked ÐAPPs - created contracts
This data is stored as an IPLD Graphs per type and stored in a users profile contract. These graphs are independant from each other and have to be saved separately.
This contract is a DataContract and can be created via the factory at profile.factory.evan and looked up at the global profile index profile.evan. The creation process and landmap looks like this:
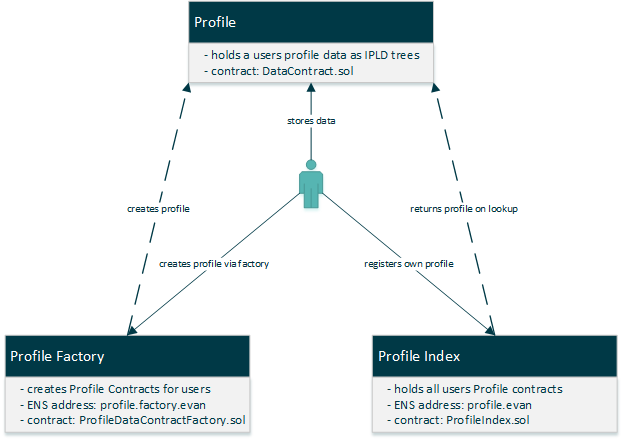
Basic Usage¶
// the bookmark we want to store
const sampleDesc = {
title: 'sampleTest',
description: 'desc',
img: 'img',
primaryColor: '#FFFFFF',
};
// create new profile, set private key and keyexchange partial key
await profile.createProfile(keyExchange.getDiffieHellmanKeys());
// add a bookmark
await profile.addDappBookmark('sample1.test', sampleDesc);
// store tree to contract
await profile.storeForAccount(profile.treeLabels.bookmarkedDapps);
constructor¶
new Profile(options);
Creates a new Profile instance.
Parameters¶
options
-ProfileOptions
: options for Profile constructoraccountId
-string
: account, that is the profile ownercontractLoader
-ContractLoader
:ContractLoader
instancedataContract
-DataContract
:DataContract
instanceexecutor
-Executor
:Executor
instanceipld
-Ipld
:Ipld
instancenameResolver
-NameResolver
:NameResolver
instancedefaultCryptoAlgo
-string
(optional): crypto algorith name fromCryptoProvider
, defaults toaes
log
-Function
(optional): function to use for logging:(message, level) => {...}
logLevel
-LogLevel
(optional): messages with this level will be logged withlog
logLog
-LogLogInterface
(optional): container for collecting log messageslogLogLevel
-LogLevel
(optional): messages with this level will be pushed tologLog
trees
-object
(optional): precached profile data, defaults to{}
Returns¶
Profile
instance
Example¶
const profile = new Profile({
accountId: accounts[0],
contractLoader,
dataContract,
executor,
ipld,
nameResolver,
});
createProfile¶
profile.createProfile(keys)
Create new profile, store it to profile index initialize addressBook and publicKey.
Parameters¶
keys
-any
: diffie hell man keys for account, created byKeyExchange
privateKey
-Buffer
: private key for key exchangepublicKey
-Buffer
: combination of shared secret and own private key
Returns¶
Promise
returns void
: resolved when done
exists¶
profile.exists();
Check if a profile has been stored for current account.
Parameters¶
options
-object
: The options used for calling
Returns¶
Promise
returns void
: true if a contract was registered, false if not
getContactKnownState¶
profile.getContactKnownState(accountId);
Check, known state for given account.
Parameters¶
accountId
-string
: account id of a contact
Returns¶
Promise
returns void
: true if known account
setContactKnownState¶
profile.setContactKnownState(accountId, contactKnown);
Store given state for this account.
Parameters¶
accountId
-string
: account id of a contactcontactKnown
-boolean
: true if known, false if not
Returns¶
Promise
returns void
: resolved when done
loadForAccount¶
profile.loadForAccount([tree]);
Load profile for given account from global profile contract, if a tree is given, load that tree from ipld as well.
Parameters¶
tree
-string
(optional): tree to load (‘bookmarkedDapps’, ‘contracts’, …), profile.treeLabels properties can be passed as arguments
Returns¶
Promise
returns void
: resolved when done
storeForAccount¶
profile.storeForAccount(tree);
Stores profile tree or given hash to global profile contract.
Parameters¶
tree
-string
: tree to store (‘bookmarkedDapps’, ‘contracts’, …)ipldHash
-string
(optional): store this hash instead of the current tree for account
Returns¶
Promise
returns void
: resolved when done
loadFromIpld¶
profile.loadFromIpld(tree, ipldIpfsHash);
Load profile from ipfs via ipld dag via ipfs file hash.
Parameters¶
tree
-string
: tree to load (‘bookmarkedDapps’, ‘contracts’, …)ipldIpfsHash
-string
: ipfs file hash that points to a file with ipld a hash
Returns¶
Promise
returns Profile
: this profile
storeToIpld¶
profile.storeToIpld(tree);
Store profile in ipfs as an ipfs file that points to a ipld dag.
Parameters¶
tree
-string
: tree to store (‘bookmarkedDapps’, ‘contracts’, …)
Returns¶
Promise
returns string
: hash of the ipfs file
Example¶
const storedHash = await profile.storeToIpld(profile.treeLabels.contracts);
= addressBook =¶
addContactKey¶
profile.addContactKey(address, context, key);
Add a key for a contact to bookmarks.
Parameters¶
address
-string
: account key of the contactcontext
-string
: store key for this context, can be a contract, bc, etc.key
-string
: communication key to store
Returns¶
Promise
returns void
: resolved when done
addProfileKey¶
profile.addProfileKey(address, key, value);
Add a profile value to an account.
Parameters¶
address
-string
: account key of the contactkey
-string
: store key for the account like alias, etc.value
-string
: value of the profile key
Returns¶
Promise
returns void
: resolved when done
Example¶
await profile.addProfileKey(accounts[0], 'email', 'sample@example.org');
await profile.addProfileKey(accounts[0], 'alias', 'Sample Example');
getAddressBookAddress¶
profile.getAddressBookAddress(address);
Function description
Parameters¶
address
-string
: contact address
Returns¶
Promise
returns any
: bookmark info
getAddressBook¶
profile.getAddressBook();
Get the whole addressBook.
Parameters¶
(none)
Returns¶
any
: entire address book
getContactKey¶
profile.getContactKey(address, context);
Get a communication key for a contact from bookmarks.
Parameters¶
address
-string`
: account key of the contactcontext
-string`
: store key for this context, can be a contract, bc, etc.
Returns¶
Promise
returns void
: matching key
getProfileKey¶
profile.getProfileKey(address, key);
Get a key from an address in the address book.
Parameters¶
address
-string
: address to look upkey
-string
: type of key to get
Returns¶
Promise
returns any
: key
removeContact¶
profile.removeContact(address);
Remove a contact from bookmarkedDapps.
Parameters¶
address
-string
: account key of the contact
Returns¶
Promise
returns void
: resolved when done
Example¶
await profile.removeContact(address);
= bookmarkedDapps =¶
addDappBookmark¶
profile.addDappBookmark(address, description);
Add a bookmark for a dapp.
Parameters¶
address
-string
: ENS name or contract address (if no ENS name is set)description
-DappBookmark
: description for bookmark
Returns¶
Promise
returns void
: resolved when done
Example¶
const bookmark = {
"name": "taskboard",
"description": "Create todos and manage updates.",
"i18n": {
"description": {
"de": "Erstelle Aufgaben und überwache Änderungen",
"en": "Create todos and manage updates"
},
"name": {
"de": "Task Board",
"en": "Task Board"
}
},
"imgSquare": "...",
"standalone": true,
"primaryColor": "#e87e23",
"secondaryColor": "#fffaf5",
};
await profile.addDappBookmark('sampletaskboard.evan', bookmark);
getDappBookmark¶
profile.getDappBookmark(address);
Get a bookmark for a given address if any.
Parameters¶
address
-string
: ENS name or contract address (if no ENS name is set)
Returns¶
Promise
returns any
: bookmark info
getBookmarkDefinition¶
profile.getBookmarkDefinition();
Get all bookmarks for profile.
Parameters¶
(none)
Returns¶
Promise
returns any
: all bookmarks for profile
removeDappBookmark¶
profile.removeDappBookmark(address);
Remove a dapp bookmark from the bookmarkedDapps.
Parameters¶
address
-string
: address of the bookmark to remove
Returns¶
Promise
returns void
: resolved when done
setDappBookmarks¶
profile.setDappBookmarks(bookmarks);
Set bookmarks with given value.
Parameters¶
bookmarks
-any
: The options used for calling
Returns¶
Promise
returns void
: resolved when done
Example¶
const bookmarks = await profile.getBookmarkDefinitions();
// update bookmarks
// ...
await profile.setDappBookmarks(bookmarks);
= contracts =¶
addContract¶
profile.addContract(address, data);
Add a contract to the current profile.
Parameters¶
address
-string
: contract addressdata
-any
: bookmark metadata
Returns¶
Promise
returns void
: resolved when done
getContracts¶
profile.getContracts();
Get all contracts for the current profile.
Parameters¶
(none)
Returns¶
Promise
returns any
: contracts info
getContract¶
profile.getContract(address);
Get a specific contract entry for a given address.
Parameters¶
address
-string
: contact address
Returns¶
Promise
returns any
: bookmark info
addBcContract¶
profile.addBcContract(bc, address, data)
Add a contract (task contract etc. ) to a business center scope of the current profile
Parameters¶
bc
-string
: business center ens address or contract addressaddress
-string
: contact addressdata
-any
: bookmark metadata
Returns¶
Promise
returns void
: resolved when done
getBcContract¶
profile.getBcContract(bc, address);
Get a specific contract entry for a given address.
Parameters¶
bcc
-string
: business center ens address or contract addressaddress
-string
: contact address
Returns¶
Promise
returns any
: bookmark info
getBcContracts¶
profile.getBcContracts(bc, address);
Get all contracts grouped under a business center.
Parameters¶
bcc
-string
: business center ens address or contract address
Returns¶
Promise
returns any
: bookmark info
removeContract¶
profile.removeBcContract(address, data);
removes a contract (task contract etc. ) from a business center scope of the current profile
Parameters¶
bc
-string
: business center ens address or contract addressaddress
-any
: contact address
Returns¶
Promise
returns void
: resolved when done
Example¶
await profile.removeBcContract('testbc.evan', '0x');
= publicKey =¶
addPublicKey¶
profile.addPublicKey(key);
Add a key for a contact to bookmarks.
Parameters¶
key
-string
: public Diffie Hellman key part to store
Returns¶
Promise
returns void
: resolved when done
getPublicKey¶
profile.getPublicKey();
Get public key of profiles.
Parameters¶
(none)
Returns¶
Promise
returns any
: public key
loadActiveVerifications¶
profile.loadActiveVerifications();
Load all verificationss that should be displayed for this profile within the ui.
Parameters¶
(none)
Returns¶
Promise
returns Array<string>
: array of topics of verificationss that should be displayed (e.g. [ ‘/company/tuev’, ‘/test/1234’ ] )
setActiveVerifications¶
profile.setActiveVerifications(bookmarks);
Save an array of active verificationss to the profile.
Parameters¶
bookmarks
-Array<string>
: bookmarks to set
Returns¶
Promise
returns void
: resolved when saving is done
Example¶
await bcc.profile.setActiveVerifications([ '/company/tuev', '/test/1234' ]);